這次是自己做的小程式,之後會再修改一些BUG,增加更多功能
例如:
1.可以刪除所有帳目資料(洗白資料檔案)
2.可以查詢特定日期的帳目
3.可以刪除特定日期的帳目
這次的程式分成4個public class 其中有自製一個ArrayList是因為在輸入、輸出物件到檔案時,會牽涉到Object型別與泛型的問題,我還沒辦法解決,所以只能自製一個ArrayList。
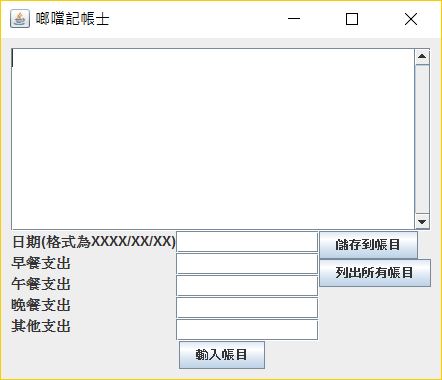
-------------------------------------------------------------------------------------------------------------------------
AccountGUI:
import java.io.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.lang.*;
public class AccountGUI {
private JFrame frame;
private JPanel panel;
private ArrayList accountList;
private Account account;
private JButton enterbutton;
private JButton displaybutton;
private JButton savebutton;
private JButton deletebutton;
private JTextArea area;
private JTextField datefield;
private JTextField breakfastfield;
private JTextField lunchfield;
private JTextField dinnerfield;
private JTextField othersfield;
private StreamHelper streamhelper;
public void buildGUI () {
frame = new JFrame("啷噹記帳士");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
accountList = new ArrayList();
streamhelper = new StreamHelper();
BorderLayout layout = new BorderLayout();
panel = new JPanel(layout);
Box rightbox = new Box(BoxLayout.Y_AXIS);
Box centerbox = new Box(BoxLayout.Y_AXIS);
Box leftbox = new Box(BoxLayout.Y_AXIS);
panel.add(BorderLayout.EAST, rightbox);
panel.add(BorderLayout.CENTER, centerbox);
panel.add(BorderLayout.WEST, leftbox);
panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
area = new JTextArea(10, 10);
area.setLineWrap(true);
area.setSelectionColor(Color.blue);
JScrollPane scroller = new JScrollPane(area);
scroller.setVerticalScrollBarPolicy(ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS);
scroller.setHorizontalScrollBarPolicy(ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
panel.add(BorderLayout.NORTH, scroller);
datefield = new JTextField(10);
breakfastfield = new JTextField(5);
lunchfield = new JTextField(5);
dinnerfield = new JTextField(5);
othersfield = new JTextField(5);
centerbox.add(datefield);
centerbox.add(breakfastfield);
centerbox.add(lunchfield);
centerbox.add(dinnerfield);
centerbox.add(othersfield);
Font font = new Font("LALA", Font.BOLD, 14);
JLabel datelabel = new JLabel("日期(格式為XXXX/XX/XX)");
datelabel.setFont(font);
JLabel breakfastlabel = new JLabel("早餐支出");
breakfastlabel.setFont(font);
JLabel lunchlabel = new JLabel("午餐支出");
lunchlabel.setFont(font);
JLabel dinnerlabel = new JLabel("晚餐支出");
dinnerlabel.setFont(font);
JLabel otherslabel = new JLabel("其他支出");
otherslabel.setFont(font);
leftbox.add(datelabel);
leftbox.add(breakfastlabel);
leftbox.add(lunchlabel);
leftbox.add(dinnerlabel);
leftbox.add(otherslabel);
enterbutton = new JButton("輸入帳目");
savebutton = new JButton("儲存到帳目");
displaybutton = new JButton("列出所有帳目");
rightbox.add(savebutton);
rightbox.add(displaybutton);
centerbox.add(enterbutton);
savebutton.addActionListener(new SaveListener());
displaybutton.addActionListener(new DisplayListener());
enterbutton.addActionListener(new EnterListener());
frame.setSize(300, 300);
frame.getContentPane().add(panel);
frame.pack();
frame.setVisible(true);
}
public class SaveListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
if (account == null) {
area.setText("錯誤!! 你沒有輸入帳目");
}
else {
accountList.add(account);
streamhelper.output(accountList);
account = null;
}
}
}
public class EnterListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
String date = datefield.getText();
String breakfast = breakfastfield.getText();
String lunch = lunchfield.getText();
String dinner = dinnerfield.getText();
String others = othersfield.getText();
datefield.setText("");
breakfastfield.setText("");
lunchfield.setText("");
dinnerfield.setText("");
othersfield.setText("");
account = new Account(Integer.parseInt(breakfast), Integer.parseInt(lunch),
Integer.parseInt(dinner), Integer.parseInt(others), date);
}
}
public class DisplayListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
accountList = streamhelper.input();
area.setText("");
for (int i = 0; i < (accountList.size()); i++) {
area.append(accountList.get(i).printAccount()+"\n");
}
}
}
public class DeleteListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
accountList = null;
streamhelper.output(accountList);
}
}
public static void main(String[] args) {
AccountGUI gui = new AccountGUI();
gui.buildGUI();
}
}
-------------------------------------------------------------------------------------------------------------------------
Account:
import java.io.*;
import java.lang.*;
public class Account implements Serializable{
private int totalExpenditureOfDay ;
private int expenditureOfDinner;
private int expenditureOfBreakfast;
private int expenditureOfLunch;
private int expenditureOfOthers;
private String date; //格式 20XX/XX/XX
public Account(int expenditureOfBreakfast, int expenditureOfLunch, int expenditureOfDinner,
int ExpenditureOfOthers, String date) {
this.expenditureOfBreakfast = expenditureOfBreakfast;
this.expenditureOfLunch = expenditureOfLunch;
this.expenditureOfDinner = expenditureOfDinner;
this.expenditureOfOthers = expenditureOfOthers;
this.date = date;
}
public int getExpenditureOfDinner() {
return expenditureOfDinner;
}
public int getExpenditureOfBreakfast() {
return expenditureOfBreakfast;
}
public int getExpenditureOfLunch() {
return expenditureOfLunch;
}
public int getExpenditureOfOthers() {
return expenditureOfOthers;
}
public int getTotalExpenditureOfDay() {
return totalExpenditureOfDay;
}
public void calculateTotalExpenditureOfDay () {
this.totalExpenditureOfDay = (expenditureOfDinner)+(expenditureOfBreakfast)+
(expenditureOfLunch)+ (expenditureOfOthers);
}
public String printAccount() {
String detail = "日期: "+date+" 早餐支出: "+Integer.toString(this.expenditureOfBreakfast)
+" 午餐支出: "+Integer.toString(this.expenditureOfLunch)+" 晚餐支出: "+Integer.toString(this.expenditureOfDinner)
+" 其他支出: "+Integer.toString(this.expenditureOfOthers);
return detail;
}
}
-------------------------------------------------------------------------------------------------------------------------
StreamHelper:
import java.io.*;
public class StreamHelper {
public void output(ArrayList output) {
try {
ObjectOutputStream os = new ObjectOutputStream(new FileOutputStream("Account.ser"));
os.writeObject(output);
} catch (IOException ex) {
ex.printStackTrace();
}
}
public ArrayList input() {
ArrayList list = null;
try {
ObjectInputStream is = new ObjectInputStream(new FileInputStream("Account.ser"));
list = (ArrayList) is.readObject();
} catch (Exception ex) {
ex.printStackTrace();
}
return list;
}
}
-------------------------------------------------------------------------------------------------------------------------
ArrayList:
import java.util.*;
import java.io.*;
public class ArrayList implements Serializable {
private Account[] elems;
private int next;
private static final long serialVersionUID = -4560544466L;
public ArrayList(int capacity) {
elems = new Account[capacity];
}
public ArrayList() {
this(50);
}
public void add (Account o) {
if (next == elems.length) {
elems = Arrays.copyOf(elems, elems.length*2);
elems[next++] = o;
}
else {
elems[next++] = o;
}
}
public Account get (int i) {
return elems[i];
}
public Account[] getElems() {
return elems;
}
public int size() {
return next;
}
}
例如:
1.可以刪除所有帳目資料(洗白資料檔案)
2.可以查詢特定日期的帳目
3.可以刪除特定日期的帳目
這次的程式分成4個public class 其中有自製一個ArrayList是因為在輸入、輸出物件到檔案時,會牽涉到Object型別與泛型的問題,我還沒辦法解決,所以只能自製一個ArrayList。
-------------------------------------------------------------------------------------------------------------------------
AccountGUI:
import java.io.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.lang.*;
public class AccountGUI {
private JFrame frame;
private JPanel panel;
private ArrayList accountList;
private Account account;
private JButton enterbutton;
private JButton displaybutton;
private JButton savebutton;
private JButton deletebutton;
private JTextArea area;
private JTextField datefield;
private JTextField breakfastfield;
private JTextField lunchfield;
private JTextField dinnerfield;
private JTextField othersfield;
private StreamHelper streamhelper;
public void buildGUI () {
frame = new JFrame("啷噹記帳士");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
accountList = new ArrayList();
streamhelper = new StreamHelper();
BorderLayout layout = new BorderLayout();
panel = new JPanel(layout);
Box rightbox = new Box(BoxLayout.Y_AXIS);
Box centerbox = new Box(BoxLayout.Y_AXIS);
Box leftbox = new Box(BoxLayout.Y_AXIS);
panel.add(BorderLayout.EAST, rightbox);
panel.add(BorderLayout.CENTER, centerbox);
panel.add(BorderLayout.WEST, leftbox);
panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
area = new JTextArea(10, 10);
area.setLineWrap(true);
area.setSelectionColor(Color.blue);
JScrollPane scroller = new JScrollPane(area);
scroller.setVerticalScrollBarPolicy(ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS);
scroller.setHorizontalScrollBarPolicy(ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
panel.add(BorderLayout.NORTH, scroller);
datefield = new JTextField(10);
breakfastfield = new JTextField(5);
lunchfield = new JTextField(5);
dinnerfield = new JTextField(5);
othersfield = new JTextField(5);
centerbox.add(datefield);
centerbox.add(breakfastfield);
centerbox.add(lunchfield);
centerbox.add(dinnerfield);
centerbox.add(othersfield);
Font font = new Font("LALA", Font.BOLD, 14);
JLabel datelabel = new JLabel("日期(格式為XXXX/XX/XX)");
datelabel.setFont(font);
JLabel breakfastlabel = new JLabel("早餐支出");
breakfastlabel.setFont(font);
JLabel lunchlabel = new JLabel("午餐支出");
lunchlabel.setFont(font);
JLabel dinnerlabel = new JLabel("晚餐支出");
dinnerlabel.setFont(font);
JLabel otherslabel = new JLabel("其他支出");
otherslabel.setFont(font);
leftbox.add(datelabel);
leftbox.add(breakfastlabel);
leftbox.add(lunchlabel);
leftbox.add(dinnerlabel);
leftbox.add(otherslabel);
enterbutton = new JButton("輸入帳目");
savebutton = new JButton("儲存到帳目");
displaybutton = new JButton("列出所有帳目");
rightbox.add(savebutton);
rightbox.add(displaybutton);
centerbox.add(enterbutton);
savebutton.addActionListener(new SaveListener());
displaybutton.addActionListener(new DisplayListener());
enterbutton.addActionListener(new EnterListener());
frame.setSize(300, 300);
frame.getContentPane().add(panel);
frame.pack();
frame.setVisible(true);
}
public class SaveListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
if (account == null) {
area.setText("錯誤!! 你沒有輸入帳目");
}
else {
accountList.add(account);
streamhelper.output(accountList);
account = null;
}
}
}
public class EnterListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
String date = datefield.getText();
String breakfast = breakfastfield.getText();
String lunch = lunchfield.getText();
String dinner = dinnerfield.getText();
String others = othersfield.getText();
datefield.setText("");
breakfastfield.setText("");
lunchfield.setText("");
dinnerfield.setText("");
othersfield.setText("");
account = new Account(Integer.parseInt(breakfast), Integer.parseInt(lunch),
Integer.parseInt(dinner), Integer.parseInt(others), date);
}
}
public class DisplayListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
accountList = streamhelper.input();
area.setText("");
for (int i = 0; i < (accountList.size()); i++) {
area.append(accountList.get(i).printAccount()+"\n");
}
}
}
public class DeleteListener implements ActionListener {
public void actionPerformed (ActionEvent ev) {
accountList = null;
streamhelper.output(accountList);
}
}
public static void main(String[] args) {
AccountGUI gui = new AccountGUI();
gui.buildGUI();
}
}
-------------------------------------------------------------------------------------------------------------------------
Account:
import java.io.*;
import java.lang.*;
public class Account implements Serializable{
private int totalExpenditureOfDay ;
private int expenditureOfDinner;
private int expenditureOfBreakfast;
private int expenditureOfLunch;
private int expenditureOfOthers;
private String date; //格式 20XX/XX/XX
public Account(int expenditureOfBreakfast, int expenditureOfLunch, int expenditureOfDinner,
int ExpenditureOfOthers, String date) {
this.expenditureOfBreakfast = expenditureOfBreakfast;
this.expenditureOfLunch = expenditureOfLunch;
this.expenditureOfDinner = expenditureOfDinner;
this.expenditureOfOthers = expenditureOfOthers;
this.date = date;
}
public int getExpenditureOfDinner() {
return expenditureOfDinner;
}
public int getExpenditureOfBreakfast() {
return expenditureOfBreakfast;
}
public int getExpenditureOfLunch() {
return expenditureOfLunch;
}
public int getExpenditureOfOthers() {
return expenditureOfOthers;
}
public int getTotalExpenditureOfDay() {
return totalExpenditureOfDay;
}
public void calculateTotalExpenditureOfDay () {
this.totalExpenditureOfDay = (expenditureOfDinner)+(expenditureOfBreakfast)+
(expenditureOfLunch)+ (expenditureOfOthers);
}
public String printAccount() {
String detail = "日期: "+date+" 早餐支出: "+Integer.toString(this.expenditureOfBreakfast)
+" 午餐支出: "+Integer.toString(this.expenditureOfLunch)+" 晚餐支出: "+Integer.toString(this.expenditureOfDinner)
+" 其他支出: "+Integer.toString(this.expenditureOfOthers);
return detail;
}
}
-------------------------------------------------------------------------------------------------------------------------
StreamHelper:
import java.io.*;
public class StreamHelper {
public void output(ArrayList output) {
try {
ObjectOutputStream os = new ObjectOutputStream(new FileOutputStream("Account.ser"));
os.writeObject(output);
} catch (IOException ex) {
ex.printStackTrace();
}
}
public ArrayList input() {
ArrayList list = null;
try {
ObjectInputStream is = new ObjectInputStream(new FileInputStream("Account.ser"));
list = (ArrayList) is.readObject();
} catch (Exception ex) {
ex.printStackTrace();
}
return list;
}
}
-------------------------------------------------------------------------------------------------------------------------
ArrayList:
import java.util.*;
import java.io.*;
public class ArrayList implements Serializable {
private Account[] elems;
private int next;
private static final long serialVersionUID = -4560544466L;
public ArrayList(int capacity) {
elems = new Account[capacity];
}
public ArrayList() {
this(50);
}
public void add (Account o) {
if (next == elems.length) {
elems = Arrays.copyOf(elems, elems.length*2);
elems[next++] = o;
}
else {
elems[next++] = o;
}
}
public Account get (int i) {
return elems[i];
}
public Account[] getElems() {
return elems;
}
public int size() {
return next;
}
}